Table of Contents
Lesson Overview
Topic: Python Programming Advanced
Activities & Due Dates: [Activity 7] If-else logig implementation
Activity 1 – For loop
•for loop: Repeats a set of statements over a group of values.
•Syntax:
for variableName in groupOfValues:
statements
•We indent the statements to be repeated with tabs or spaces.
•variableName gives a name to each value, so you can refer to it in the statements. •groupOfValues can be a range of integers, specified with the range function.
•Example:
for x in range(1, 6):
print (x, “squared is”, x * x)
Output:
1 squared is 1
2 squared is 4
3 squared is 9
4 squared is 16
5 squared is 25
Activity 2 – Cumulative loops
•Some loops incrementally compute a value that is initialized outside the loop. This is sometimes called a cumulative sum.
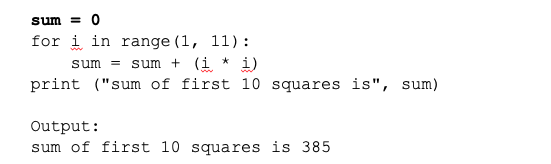
Activity 3 – if Statement
•if statement: Executes a group of statements only if a certain condition is true. Otherwise, the statements are skipped.
•Syntax:
if condition:
statements
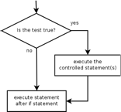
•Example:
gpa = 3.4
if gpa > 2.0:
print (“Your application is accepted.“)
Activity 4 – if/else statement
•if/else statement: Executes one block of statements if a certain condition is True, and a second block of statements if it is False.
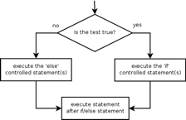
•Syntax:
if condition:
statements
else:
statements
•Example:
gpa = 1.4
if gpa > 2.0:
print (“Welcome to Mars University!“)
else:
print (“Your application is denied.“)
•Multiple conditions can be chained with elif (“else if”):
if condition:
statements
elif condition:
statements
else:
statements
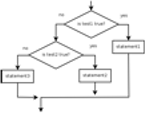
Activity 5 – Your task
•Create a grading program that assesses students’ grade using below grading system. The program needs to get a students score through console and print the grading.
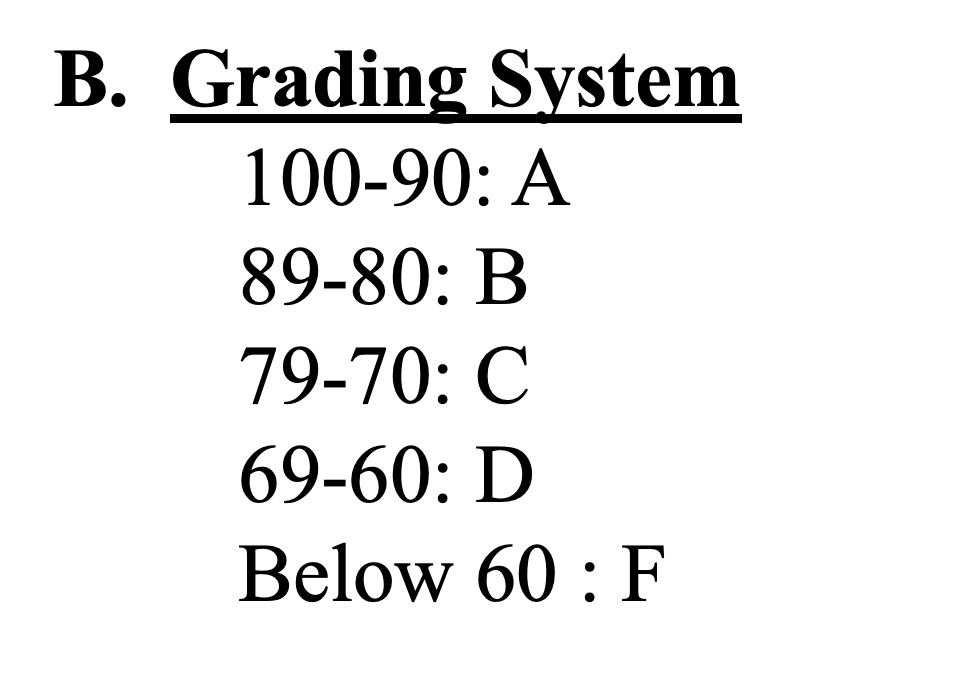
•E.g. “Enter your score: ”
•“Congratulations! Your grade is A”
•Hint: use print (), input (), and
conditional operators if, elif, and else
Activity 6 -While statement
•while loop: Executes a group of statements as long as a condition is True.
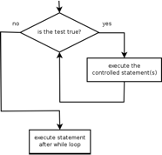
•good for indefinite loops (repeat an unknown number of times)
• Syntax:
while condition:
statements
•Example:
number = 1
while number < 200:
print (number)
number = number * 2
•Output:
1 2 4 8 16 32 64 128
Activity 7 -Logic operations in Python
•Many logical expressions use relational operators:

•Logical expressions can be combined with logical operators:
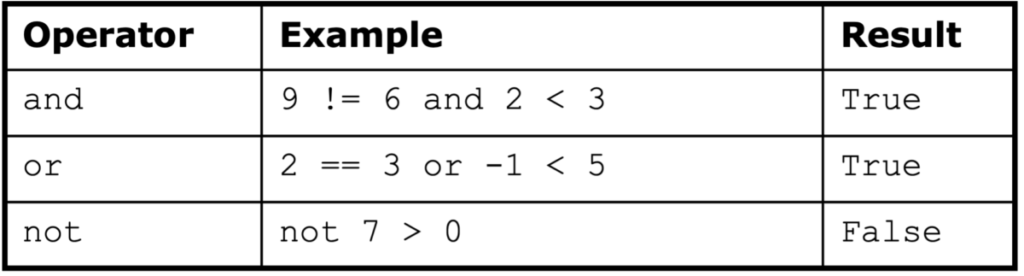
•Exercise: Write code to display and count the factors of a number.
Activity 8 -Graphics with turtle
•a popular way for introducing programming to kids
•was part of the original Logo programming language developed by Wally Feurzig and Seymour Papert in 1966
•Imagine a robotic turtle
•starting at (0, 0) in the x-y plane
•command turtle.forward(15), and it moves 15 pixels in the direction
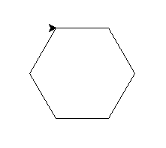
Activity 9 -Turtle Example 1: Draw a line
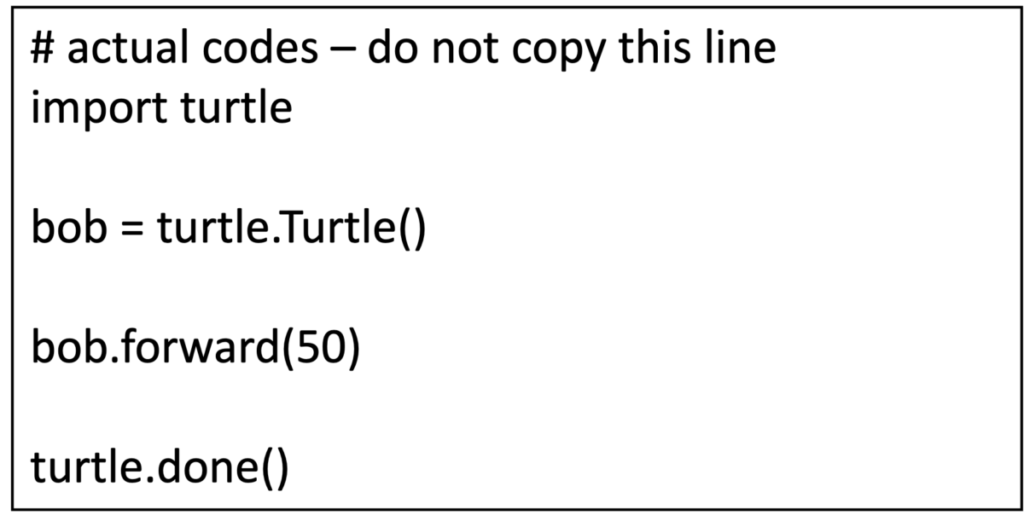
1.Import the turtle module.
2.Create a turtle to control.
3.Draw things.
4.Run turtle.done().
Activity 10 –Example 2: Drawing a square.
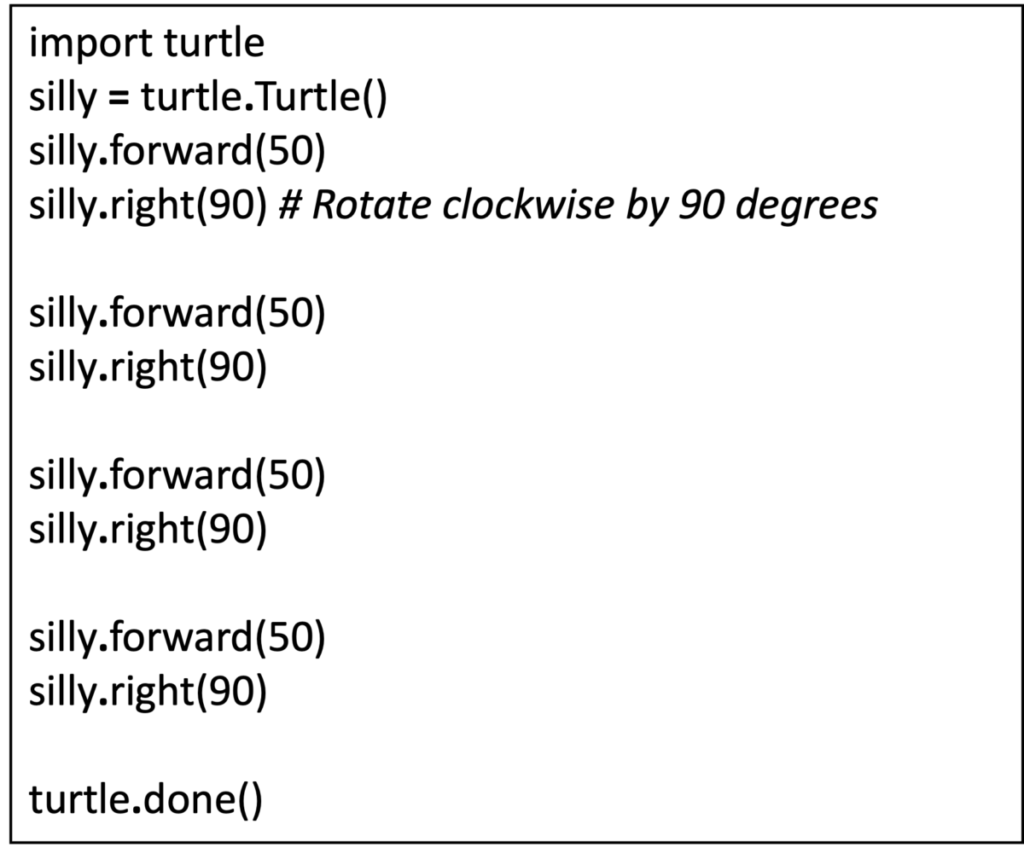
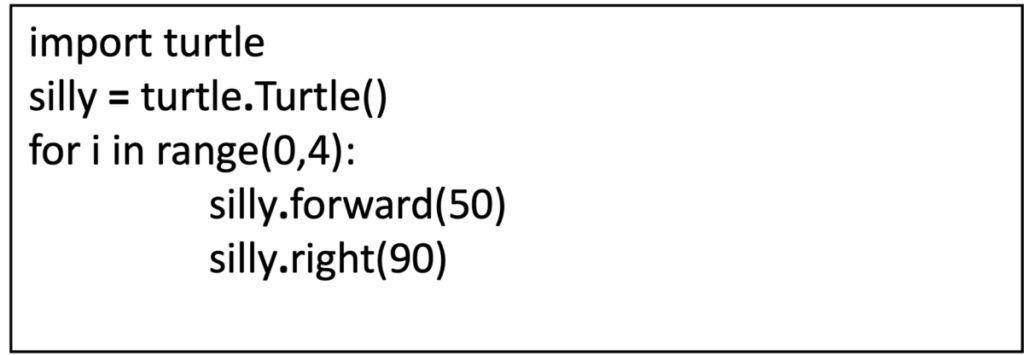
Activity 11 –Example 3: Drawing a star
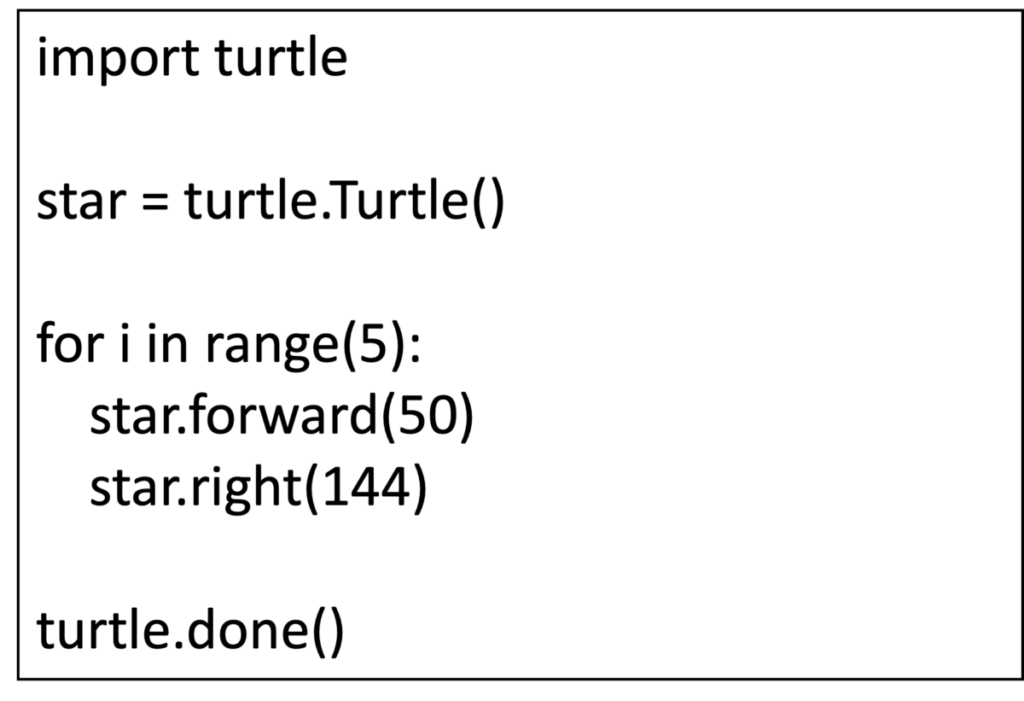
Activity 12 –Example 4: Changing line color
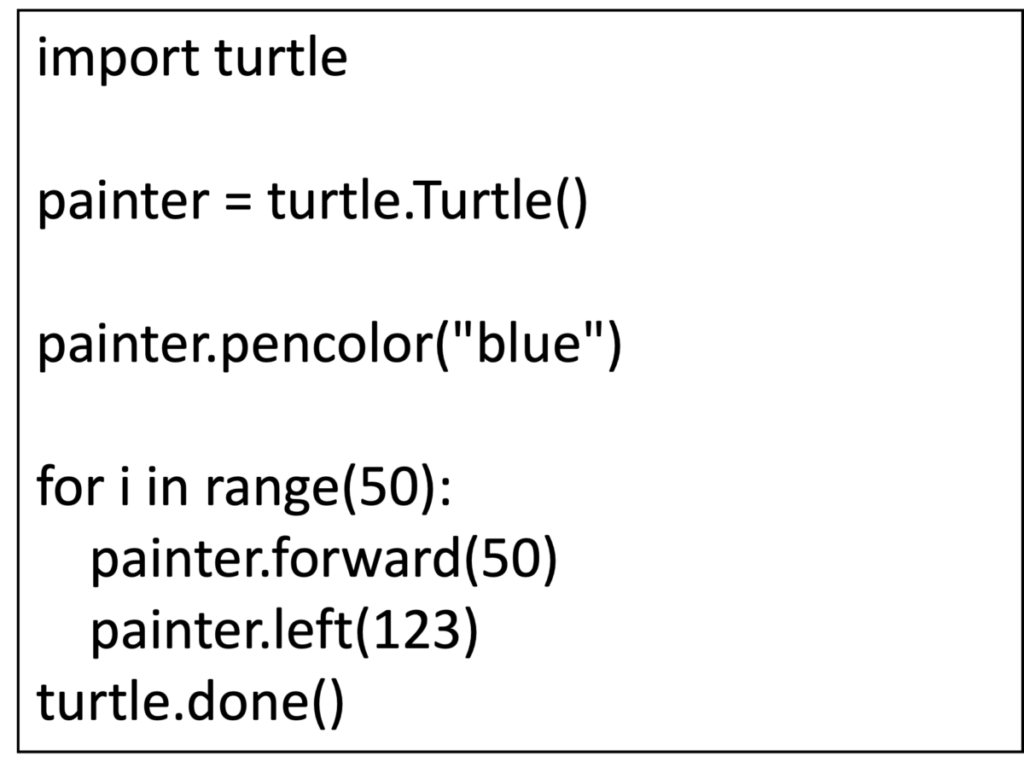
Change the pen color to another random color.
Also if you want to change the size of object, which value do you need to change?
Activity 13 -Turtle Design task
•Draw a creative graphic image using Turtle.
•You can learn more Turtle commands on Turtle Tutorial.
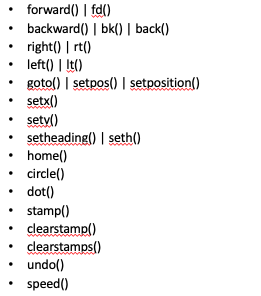