Table of Contents
Lesson Overview
Topic: Introduction to Python Programming
Activities & Due Dates: [Activity 6] Hello World in Python
Opening – Rethinking: what is the computer?
a simple machine that receives inputs, processes, and shows outcomes.

Content 1 – Evaluation of computer languages

Content 2 – A brief history of programming language
- Some influential ones:
- FORTRAN
- science/engineering
- COBOL
- business data
- LISP
- logic and AI
- BASIC
- a simple language
- FORTRAN

Activity 1 – Setting up programming environment in Raspberry Pi
1. Move to MyProgramms folder

2. Check Hello.c file and close
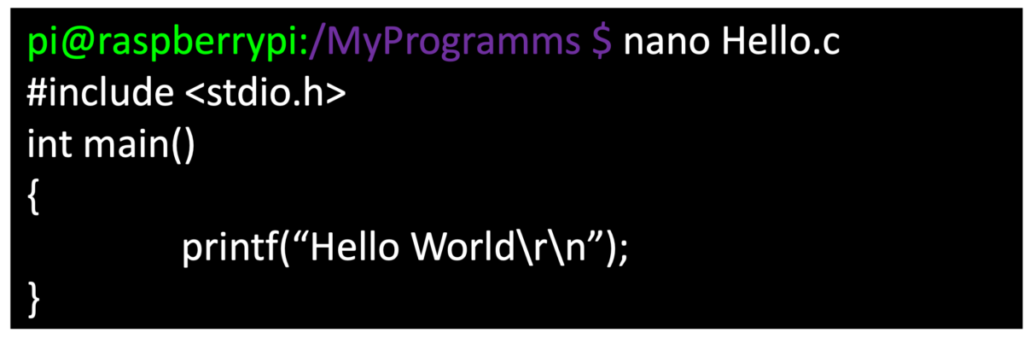
3. Generate Assembly file and compare with Hello.c

Content 3 – What is Python?
- Created by Guido van Rossum in 1991
- A programming language
- interpreted
- high-level
- general-purpose – basic computing, game, business, graphics, and all.
- Python features
- 1. Easy to learn and use
- 2. Expressive language (understandable and readable)
- 3. Interpreted language (execute line by line – easy to debug)
- 4. Cross-platform
- 5. Free and open source
- 6. Object-oriented
- 7. Extensible (Easily integrated with languages like C, Java, and C++)
- 8. Large Standard Library
- 9. GUI Programming Support
Content 4 – Programming basics
•code or source code: The sequence of instructions in a program.
•syntax: The set of legal structures and commands that can be used in a particular programming language.
•output: The messages printed to the user by a program.
•console: The text box onto which output is printed.
•Some source code editors pop up the console as an external window, and others contain their own console window.
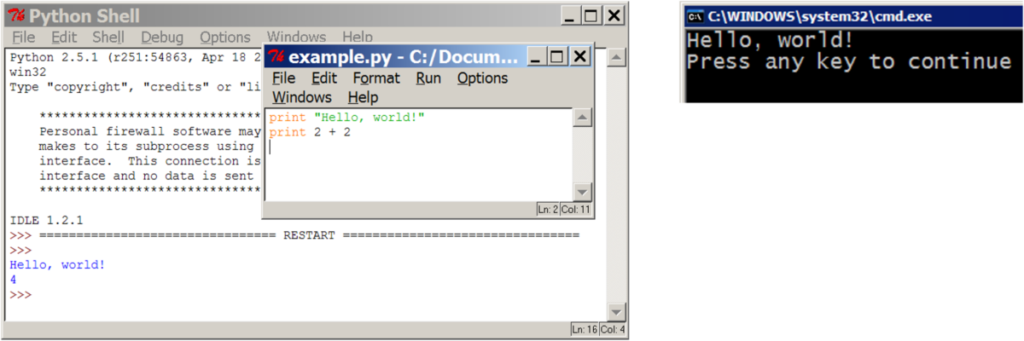
Content 5 – Compiling and interpreting
•Many languages require you to compile (translate) your program into a form that the machine understands.

•Python is instead directly interpreted into machine instructions.

Activity 2 – Running Python on Raspberry Pi
•Thonny Python IDE

Activity 3 – Python Expressions
•expression: A data value or set of operations to compute a value.
Examples: 1 + 4 * 3
•Arithmetic operators we will use:
+ – * / addition, subtraction/negation, multiplication, division
% modulus, a.k.a. remainder
** exponentiation
•precedence: Order in which operations are computed.
* / % ** have a higher precedence than + –
1 + 3 * 4 is 13
•Parentheses can be used to force a certain order of evaluation.
(1 + 3) * 4 is 16
Activity 4 – Real numbers in Python
•Python can also manipulate real numbers.
Examples: 6.022 -15.9997 42.0 2.143e17
•The operators + – * / % ) all work for real numbers.
•The / produces an exact answer: 15.0 / 2.0 is 7.5
•The same rules of precedence also apply to real numbers:
Evaluate ( ) before * / % before + –
•When integers and reals are mixed, the result is a real number.
Example: 1 / 2.0 is 0.5
The conversion occurs on a per-operator basis.
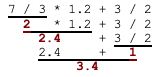
Activity 5 – Variables
- variable: A named piece of memory that can store a value.
- Usage:
- Compute an expression’s result,
- store that result into a variable,
- and use that variable later in the program.
- assignment statement: Stores a value into a variable.
- Syntax:
name = value
Examples: x = 5
gpa = 3.14

•A variable that has been given a value can be used in expressions.
x + 4 is 9
Activity 6 – Print out a message in Python
•print : Produces text output on the console.
•Syntax:
print (“Message“)
print (Expression)
•Prints the given text message or expression value on the console, and moves the cursor down to the next line.
print (Item1, Item2, …, ItemN)
•Prints several messages and/or expressions on the same line.
•Examples:
print (“Hello, world!“)
age = 45
print (“You have”, 65 – age, “years until retirement“)
Output:
Hello, world!
You have 20 years until retirement
Activity 7 – Data types
•Numeric
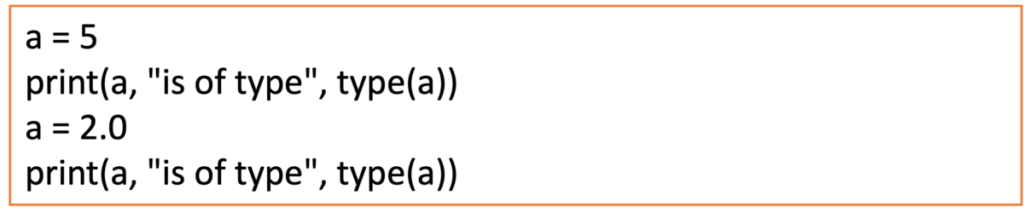
•Integer
•Float
•Strings
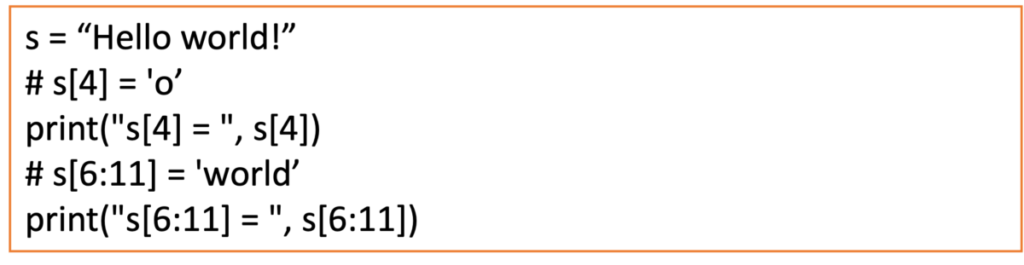
•And others: Boolean, Date, List …
Activity 8 – input
•input : Reads a number from user input.
•You can assign (store) the result of input into a variable.
•Example:
age = input(“How old are you? “)
print (“Your age is”, age)
print (“You have”, 65 – int(age), “years until retirement“)
Output:
How old are you? 53
Your age is 53
You have 12 years until retirement
•Exercise: Write a Python program that prompts the user for his/her amount of money, then reports how many Nintendo Switches the person can afford, and how much more money he/she will need to afford an additional Switches. (Assume a Nintendo Switch is $299)
•hint: use / and % operators
•Convert input value using int(input), because the input value is a text variable.