In making Project Epsilon, coding took up the vast majority of time. In fact, it took more time than design and asset collection combined.
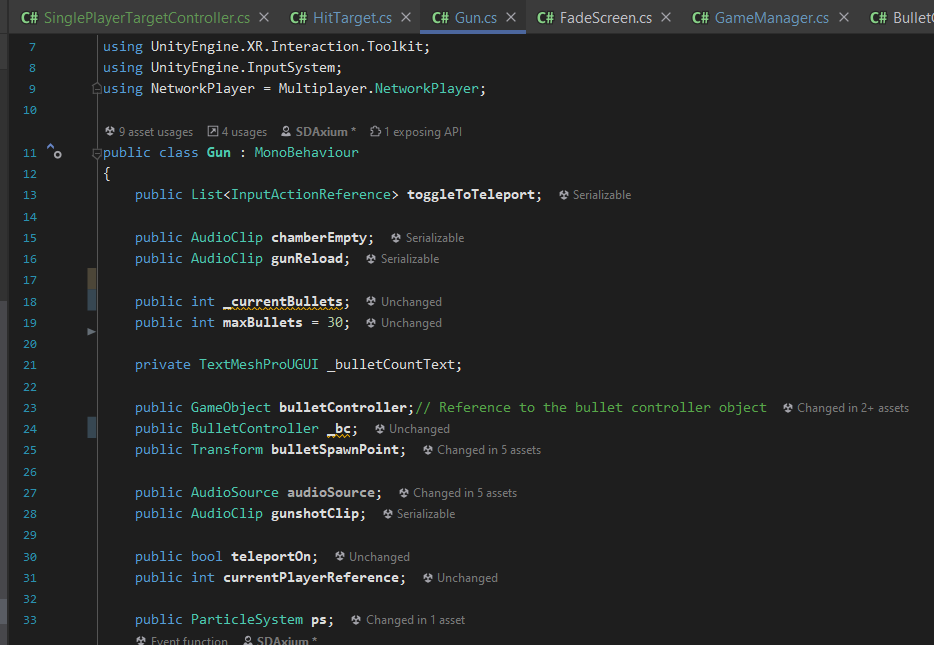
The gun is the most important part of the game. The player by the end of the game can do almost nothing without it. Despite being so important, the gun is also simple. You have a gun, you can shoot with it. In the beginning the only variables on the gun were: chamberEmpty, gunReload, gunshotClip, currentBullets, maxBullets, and bulletSpawnPoint.
Chamber empty, gun reload and gunshot clip are all sounds. Chamber empty played if the player attempted to fire with no bullets. Gun reload played when the player reloaded their gun. Gunshot clip played whenever the player fired a bullet. Current bullets showed how many times the player could shoot before having to reload. Max bullets showed how the starting number of bullets in a gun as well as the number of bullets the gun would go back to on reload.
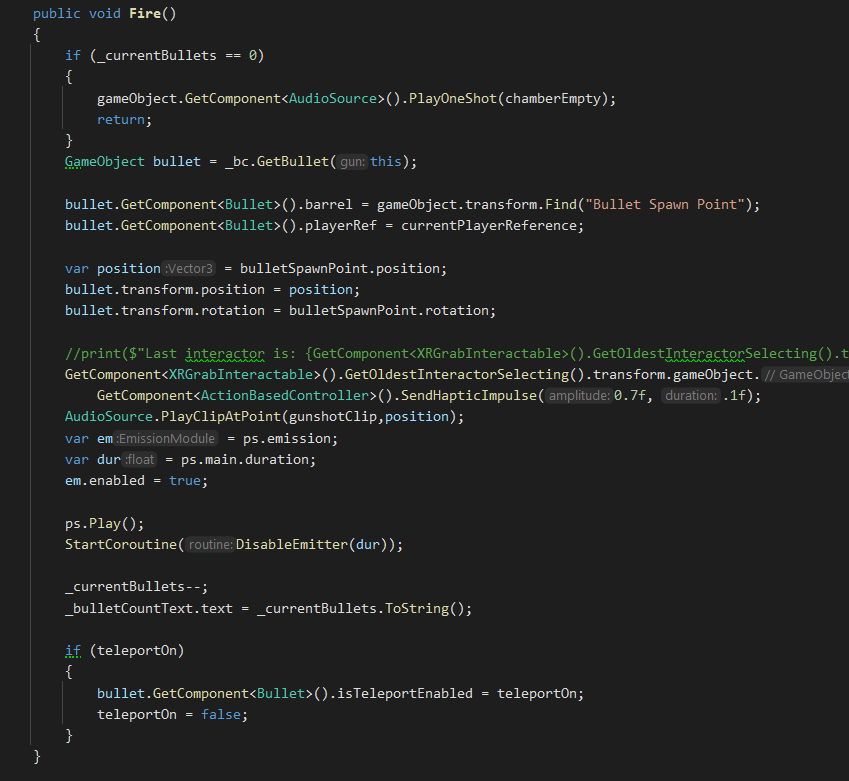
Any functional gun can fire. The fire script started off as a simple method that played a sound(gunshot or chamber empty), instantiated a bullet, and sent the bullet flying. As the semester progressed, the firing of the bullet also became more complicated. The sound played remained the same but the gun no longer made its own bullet
An early optimization done for bullets was to remove the constant spawning. Bullets would be fired near endlessly and it isn’t good practice to repeatedly spawn objects. Instead, all guns now had a reference to the BulletController, a manager which held all the bullets in the game, handing them to different guns as needed and spawning new bullets if necessary.
When the teleportation system provided by the XR interaction toolkit failed to meet all the requirements needed by my game, the matter of teleportation was handed over to the bullet and gun. This made it so the gun. which previously had no use for knowing which game object was currently holding it, suddenly did need to know. The reference to the player would then be passed onto any bullets fired from the gun, and later used by bullets to allow teleportation.
A cool thing discovered later on is that the XRGrabInteractable, the component that allows the player to pick up objects(such as a gun) actually had a reference to anything interacting with it. This allowed me to send haptic feedback whenever the player fired a bullet.

The main function of the bullet controller script was to have a simple way of managing every bullet in the game. Bullets, along with targets, are never actually destroyed in Epsilon. They are only deactivated until next needed. Gun that fire ask the bullet controller for a bullet, and if there are any inactive bullets, the controller gives it to the gun, setting up the bullet to properly behave with said gun. If there are no inactive bullets, a new one is made.
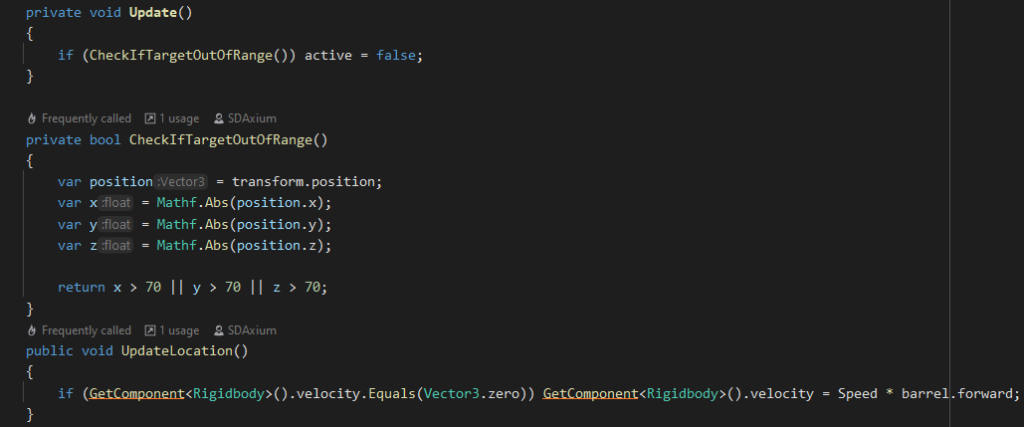
Active bullets are moved to a new list and remain there until they hit something or travel too far away from the player that shot them. The active bullets list calls the UpdateLocation() method on all bullets, moving them straight forward from where they were fired.