Description
In lab 6 we’re given an interface Movable Object. Therefore, base on this program we had to create three different classes with names of Car, Plane, Ship to implement it to the Interface Mobable object. Moreover, we had to create a program that runs an array that will call the entire classes and methods giving a desired output, this has to be done in the polymorphous technique..
Codes
Interface Movable
//Tax, Julio public interface Movable { public void moveForward(); public void moveBackward(); public void stop(); public void moveLeft(); public void moveRight(); }
Planes
//Tax, Julio public class planes implements Movable { public static void main(String[] args) { } public void moveForward() { System.out.println("Plane is flying forward to New York City"); } public void moveBackward() { System.out.println("Plane is flying backwards to Brazil"); } public void stop() { System.out.println("This plane is not flying"); } public void moveLeft() { System.out.println("Planes is turning left to go to Brazil"); } public void moveRight() { System.out.println("Planes is going right to go to NYC"); } }
Car
//Tax, Julio public class car implements Movable { public static void main(String[] args) { } public void moveForward() { System.out.println("Car is driving forward to Ohio); } public void moveBackward() { System.out.println("Car is driving Boston"); } public void stop() { System.out.println("Car is parked"); } public void moveLeft() { System.out.println("Car is turning left to interccesion"); } public void moveRight() { System.out.println("Car is turning right to the right plane"); } }
Ships
//Tax Julio public class ships implements Movable { public static void main(String[] args) { } public void moveForward() { System.out.println("ship is going forward to Mexico"); } public void moveBackward() { System.out.println("ship is going backward to NYC"); } public void stop() { System.out.println("ship is not moving"); } public void moveLeft() { System.out.println("ship is turning left"); } public void moveRight() { System.out.println("ship is turning right"); } }
MovableApp
//Tax, Julio public class MovableApp { public static void main(String[] args) { Movable[] myMovables = new Movable[3]; myMovables[0] = new car(); myMovables[1] = new planes(); myMovables[2] = new ships(); for(Movable m: myMovables){ m.moveForward(); m.moveBackward(); m.stop(); m.moveLeft(); m.moveRight(); System.out.printf(""); } } }
Screenshots
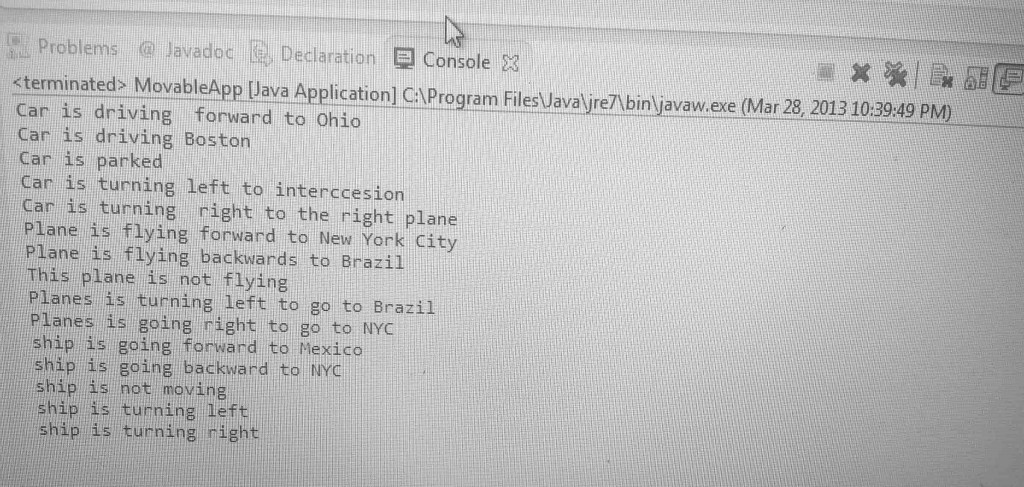