Description
The main objective for this lab was to get familiar with the syntax of Java programming. For instance by doing this arithmetic solver program we need to have a considerable knowledge on java structures such as; classes, if statement, and while statement. Furthermore, by doing this lab there’s a need to use the import.java.util.scanner keyword which is very useful when it comes to breaking down formatted input into tokens and translating individual tokens according to their data type by the use of Scanner. In consequence, by undersating all these components it was posible to create this type of program that was required in the lab.
// lab2 by Julio Tax import java.util.Scanner; public class Lab2 { public static void main(String[] args) { int number =1; int max = 0; int min = 1; int totalNum = 0; int counter = 1; int numberAmount = 0; double average; int firstdigit = number; int lastdigit = number % 10; Scanner input = new Scanner(System.in); System.out.println("enter a series of numbers."); System.out.println("enter 0 when finished numbers"); do{ number=input.nextInt(); numberAmount ++; totalNum = totalNum + number; if (number > max) { max=number; counter=0; } if(number==max) counter++; else if (number < min) { min=number; counter=1; } if(number==min) counter ++; } while (number !=0); average=(double)totalNum/(numberAmount-1); System.out.printf("The average is %.2f\n", average); System.out.println("the total number you entered: " + numberAmount); System.out.println("The sum for the numbers you entered is " + totalNum); System.out.println("The maximum number is: " + max); System.out.println("The minimum number is: " + min); firstdigit= counter-totalNum; System.out.printf("the first digit you entered is ." + firstdigit); } }
Screenshots
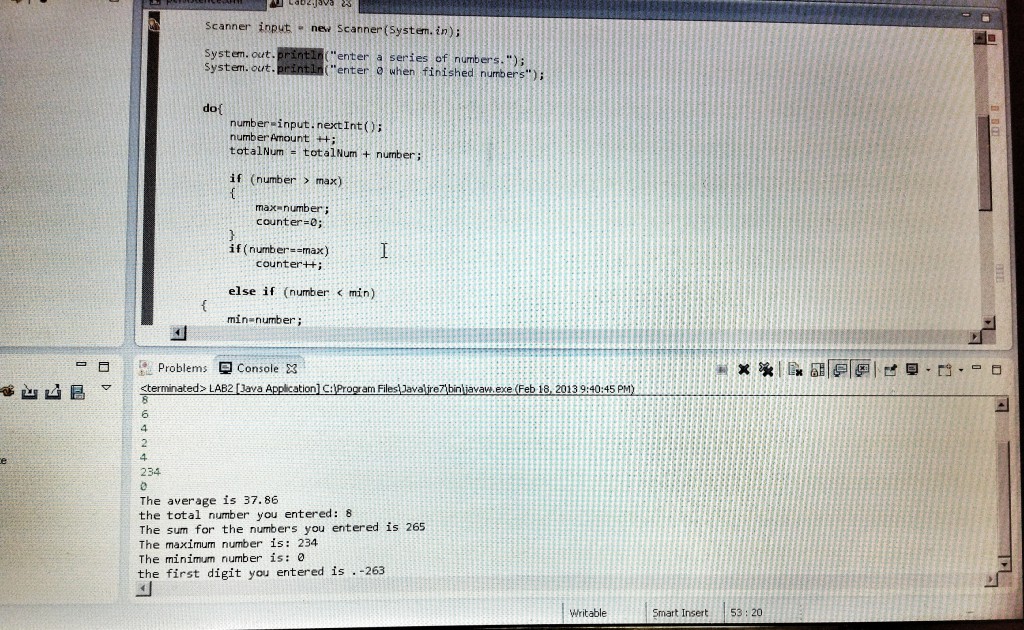